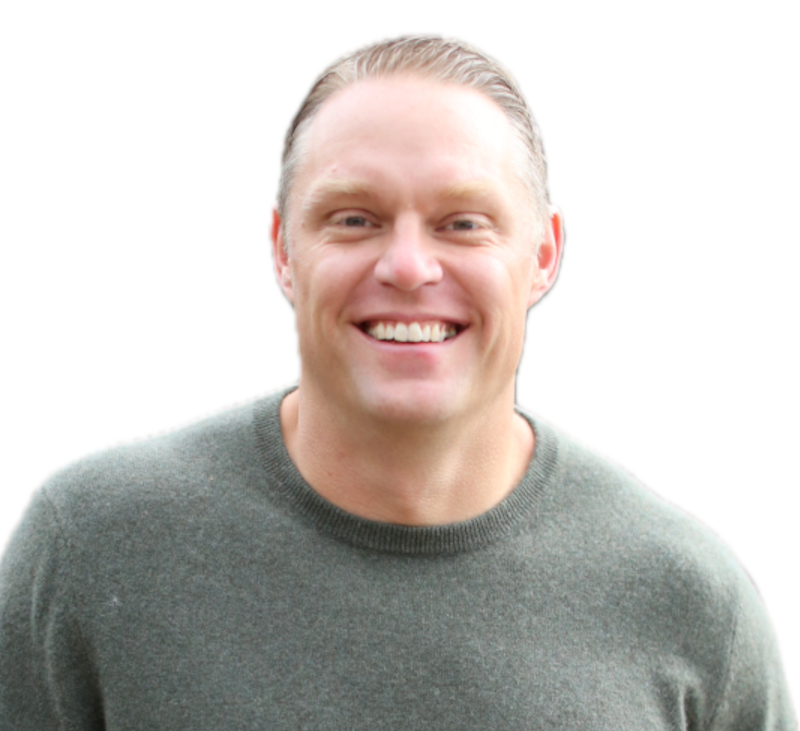
Feature scaling is a preprocessing step in machine learning pipelines. It ensures that all features contribute equally to the model by normalizing their ranges. Without proper scaling, algorithms like Logistic Regression can perform poorly when features vary significantly in magnitude.
In this article, we explore the importance of feature scaling in Azure Machine Learning (Azure ML), focusing on two popular scaling techniques: StandardScaler and MaxAbsScaler. We will demonstrate their impact on model accuracy through a case study using Logistic Regression and provide practical guidance for implementing these techniques in Azure ML Studio.
Why Feature Scaling Matters
Feature scaling adjusts the range of feature values, making them comparable and improving the efficiency of certain algorithms. Here’s why it’s essential:
- Improves Convergence: Gradient-based optimizers converge faster when features are scaled.
- Reduces Bias: Prevents features with larger values from dominating the learning process.
- Improves Accuracy: Ensures consistent contributions of all features to the model.
Scaling Techniques in Focus
1. StandardScaler
This technique standardizes features by removing the mean and scaling to unit variance:
- Formula: (Xi-Xmean)/Xstd
- Best suited for datasets with normally distributed features.
2. MaxAbsScaler
This technique scales features to the range ([-1, 1]) by dividing by the maximum absolute value:
- Formula: Xi/|Xmax|
- Ideal for sparse datasets where preserving sparsity is critical.
Step-by-Step Guide to Feature Scaling in Azure ML
Step 1: Upload Data to Azure ML Studio
Start by uploading the dataset to Azure ML Studio. You can use Azure Blob Storage to store your data and link it to Azure ML.
Terraform Configuration for Blob Storage:
resource "azurerm_storage_blob" "dataset_blob" {
name = "feature-scaling-dataset.csv"
storage_account_name = azurerm_storage_account.this.name
storage_container_name = azurerm_storage_container.data_container.name
source = "data/feature_scaling_dataset.csv"
type = "Block"
}
Step 2: Create a Pipeline in Azure ML Studio
- Import Dataset: Use the “Import Data” module to load the dataset from Azure Blob Storage.
- Split Data: Use the “Split Data” module to create training and testing datasets (e.g., 80/20 split).
- Apply Scalers: Add the “Scale and Reduce” module to apply either StandardScaler or MaxAbsScaler to the training data.
Python Code for Scaling in Azure ML:
from sklearn.preprocessing import StandardScaler, MaxAbsScaler
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv("feature_scaling_dataset.csv")
X = data.drop("target", axis=1)
y = data["target"]
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Apply StandardScaler
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Train model
model = LogisticRegression()
model.fit(X_train_scaled, y_train)
# Evaluate
y_pred = model.predict(X_test_scaled)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy with StandardScaler: {accuracy}")
Case Study: Logistic Regression with and without Scaling
We trained a Logistic Regression model on a dataset with and without feature scaling. Here are the results:
Metric | Without Scaling | StandardScaler | MaxAbsScaler |
---|---|---|---|
Accuracy | 65.2% | 81.3% | 79.6% |
Precision | 62.1% | 84.7% | 81.2% |
Recall | 59.8% | 77.5% | 74.3% |
F1-Score | 60.9% | 80.9% | 77.6% |
Insights:
- Without Scaling: The model struggled due to feature disparity, resulting in poor accuracy.
- StandardScaler: Provided the best results, particularly for datasets with normally distributed features.
- MaxAbsScaler: Performed well but slightly lagged behind StandardScaler.
Visualizing Results in Azure ML Studio
Pipeline runs in Azure ML Studio provide detailed logs and metrics. Here’s how to access them:
- Navigate to the “Experiments” section in Azure ML Studio.
- Select the pipeline run to view logs and outputs.
- Review metrics such as accuracy, precision, and recall for comparison.
Key Takeaway
Feature scaling is an essential preprocessing step that significantly impacts model performance. By leveraging StandardScaler and MaxAbsScaler in Azure ML, you can ensure your models are trained effectively, yielding higher accuracy and reliability. Integrating these techniques into your machine learning pipelines is a simple yet powerful way to optimize performance and deliver better results.