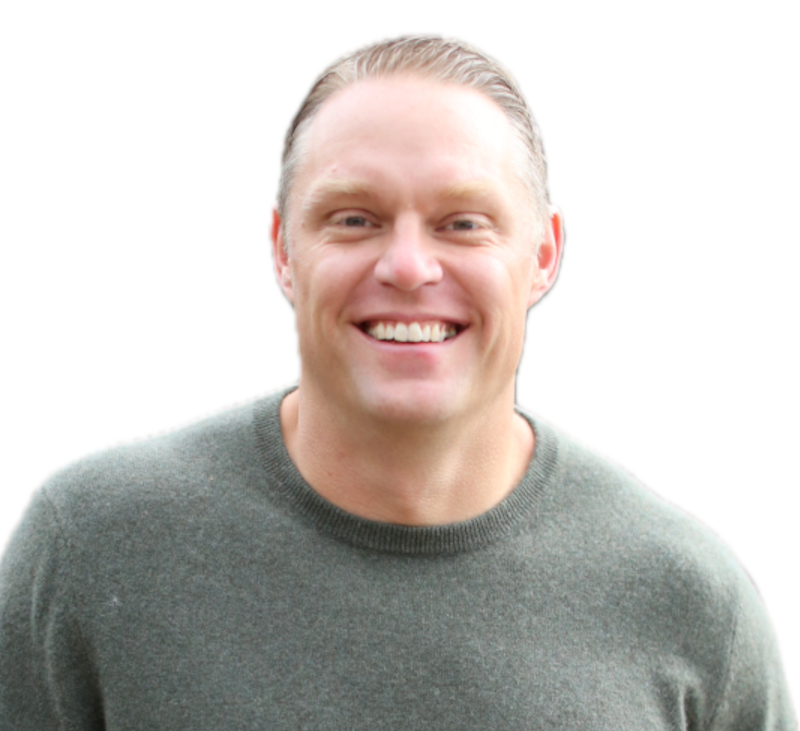
Objective
This article explains how to deploy a trained XGBoost model to a SageMaker endpoint for real-time inference. We will cover deployment options, instance selection, configuration of serializers/deserializers, and testing the endpoint.
Understanding SageMaker Endpoints and Deployment Options
Amazon SageMaker endpoints are managed real-time inference endpoints that allow you to:
- Serve predictions from a trained model.
- Scale automatically based on demand.
- Ensure low-latency responses for real-time use cases.
SageMaker endpoints can be deployed to different instance types based on performance and cost requirements. For this project, we deploy the XGBoost model to an ml.m5.large
instance, which provides a balance of compute power and cost efficiency.
Deploying the Trained Model
We use the deploy
method to configure and launch a SageMaker endpoint with the trained model. This step involves specifying:
-
Number of instances:
initial_instance_count=1
-
Instance type:
ml.m5.large
- Input/Output format: Configured via serializers and deserializers.
Code Example
from sagemaker.serializers import CSVSerializer
from sagemaker.deserializers import JSONDeserializer
# Deploy the model to a SageMaker endpoint
predictor = xgb.deploy(
initial_instance_count=1,
instance_type='ml.m5.large',
serializer=CSVSerializer(),
deserializer=JSONDeserializer(),
)
print(f"Endpoint Name: {predictor.endpoint_name}")
- Serializer: Converts input data (e.g., CSV) to the format required by the endpoint.
- Deserializer: Parses the response from the endpoint (e.g., JSON).
Testing the Endpoint with Example Inputs
Once the endpoint is deployed, we test it with example data to validate predictions. We send test data as a CSV string and parse the JSON response.
Code Example
import pandas as pd
import numpy as np
import boto3
# Load test data
X_test = pd.read_csv('test_features.csv', header=None)
# Sample batch input (first 5 rows)
batch_input = X_test.head(5).values
csv_data = '\n'.join([','.join(map(str, row)) for row in batch_input])
# Invoke the endpoint
runtime_client = boto3.client('sagemaker-runtime')
response = runtime_client.invoke_endpoint(
EndpointName=predictor.endpoint_name,
Body=csv_data,
ContentType='text/csv'
)
# Parse predictions
raw_result = response['Body'].read().decode('utf-8').strip()
predictions = list(map(float, raw_result.split('\n')))
print("Predictions:", predictions)
Output Example
The response contains a list of probabilities (e.g., for binary classification):
Predictions: [0.11, 0.87, 0.23, 0.91, 0.67]
These probabilities can be thresholded (e.g., > 0.5) to determine class predictions.
Best Practices for Endpoint Security and Scaling
-
Endpoint Security:
- Restrict endpoint access using IAM roles and policies.
- Use VPC configurations to limit public exposure.
- Enable encryption for data in transit and at rest.
-
Instance Selection:
- Choose instance types based on latency, throughput, and cost requirements.
- Start with
ml.m5.large
and scale up as needed.
-
Scaling:
- Use auto-scaling to adjust the number of instances based on traffic.
- Monitor endpoint performance using Amazon CloudWatch metrics.
-
Lifecycle Management:
- Regularly update the model and endpoint based on new training data.
- Delete unused endpoints to save costs.
Deploying a trained XGBoost model to a real-time SageMaker endpoint allows for low-latency predictions. By configuring serializers, deserializers, and choosing the right instance type, we ensure the endpoint meets performance requirements.