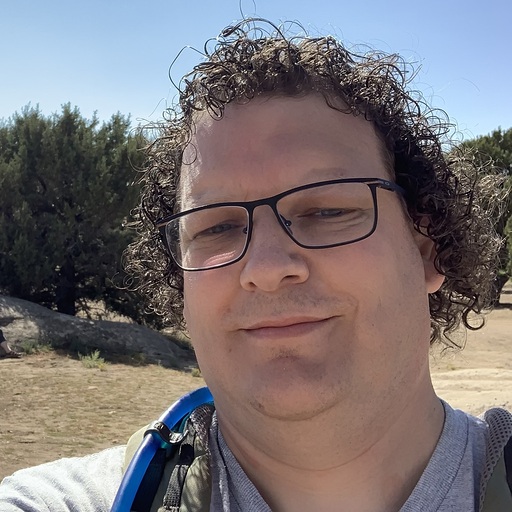
Introduction
This guide will show you how to build and deploy a simple 3-Tier application using Amazon Web Services (AWS) Serverless architecture using Terraform.
Serverless architecture is a software design pattern that allows developers to build and run applications and services without managing the underlying infrastructure. The cloud provider or third-party service manages the infrastructure in a serverless architecture. It automatically allocates resources as needed, allowing developers to focus on writing code and delivering business value.
In a 3-tier application architecture, Amazon API Gateway handles incoming HTTP requests, AWS Lambda functions serve as the application's business logic, and Amazon DynamoDB stores and retrieves data. This serverless architecture is highly scalable, cost-effective, and easy to maintain.
A 3-tier application solution is a widely-used architecture pattern that divides an application into three logical layers or tiers designed to separate concerns, improve maintainability, and simplify scaling. The three tiers include the following:
Presentation Tier (Frontend): This tier is the user interface layer that users interact with, often built using HTML, CSS, JavaScript, or frontend frameworks like React, Angular, or Vue.js. This tier displays data, captures user input, and sends requests to the backend.
Application Tier (Business Logic): This layer, also known as the business logic or service layer, processes user requests, manages application data, enforces business rules, and performs necessary computations. It typically consists of server-side programming languages, such as Python, Node.js, Java, or .NET, and can include APIs, microservices, or serverless functions like AWS Lambda.
Data Tier (Data): The data tier, or the database or storage layer, is responsible for storing and managing application data. It typically includes relational databases like MySQL, PostgreSQL, SQL Server, or NoSQL databases like MongoDB or DynamoDB and handles tasks like data retrieval, storage, and manipulation.
In 3-tier architecture, the tiers communicate with each other through well-defined interfaces, which helps promote modularity, maintainability, and scalability. The separation of concerns allows developers to update or modify one tier without affecting the others, making it easier to adapt to changing requirements and technologies.
Using AWS Serverless technology offers several benefits for developers and businesses, enabling them to build and run applications without managing the underlying infrastructure. Key reasons to use AWS Serverless technology include the following:
Cost-efficiency: Serverless applications only consume resources when they are actively running. You pay for the compute resources you use rather than pre-allocated capacity, which can result in cost savings.
Scalability: AWS Serverless technology automatically scales your applications based on the number of incoming requests or events. This eliminates manual scaling and ensures your applications can efficiently handle varying workloads.
Operational simplicity: With serverless, you no longer need to manage, patch, or monitor servers. AWS controls the infrastructure, allowing you to focus on your application code and functionality.
Faster time-to-market: Serverless architectures enable rapid application development and deployment. By focusing on the application code and leveraging managed services, you can bring your applications to market more quickly.
Flexibility: AWS Serverless technology supports many use cases, including web applications, data processing pipelines, and event-driven architectures. You can build serverless applications using various programming languages like Python, Node.js, Java, and more.
High availability: AWS ensures high availability and fault tolerance for your serverless applications by automatically distributing them across multiple availability zones.
Integrated ecosystem: AWS Serverless technology integrates seamlessly with other AWS services, such as API Gateway, DynamoDB, S3, and more, making it easy to build end-to-end serverless applications.
What we are going to use
Infrastructure as Code (IaC)is the practice of defining, provisioning, and managing the infrastructure through code instead of manual processes. IaC allows developers and operations teams to write code in a declarative language that automates the process of provisioning and managing servers, networks, databases, and other infrastructure components. This approach can make infrastructure management more efficient, reliable, scalable, and more easily repeatable and auditable.
Terraformfrom HashiCorp is an open-source Infrastructure as Code (IaC) cross-platform tool. We will be utilizing it as our Infrastructure as Code toolset. Terraform lets you define and manage infrastructure declaratively instead of manually configuring infrastructure through the console, the CLI, or shell scripts.
Amazon API Gatewayis a fully managed service AWS provides. It allows you to quickly and easily create, deploy, manage, monitor, and secure REST, HTTP, and WebSocket APIs.
AWS Lambdais a serverless computing service provided by AWS. It lets you quickly build, develop, and run your code without provisioning or managing servers.
Amazon DynamoDB is a fully managed NoSQL Database provided by AWS. It is mainly a key-value database that provides fast and predictable performance.
Amazon Route 53 is a highly available and scalable Domain Name System (DNS) service. DNS translates domain names into IP addresses, allowing you to access web resources by human-readable names instead of IP addresses.
Amazon Certificate Manager (ACM) is a service that lets you easily manage, deploy, and renew Secure Sockets Layer/Transport Layer Security (SSL/TLS) certificates.
Getting Started
For this PoC, I will use Terraform to deploy the necessary AWS resources and services. You can access the git repository here. https://github.com/junglekid/aws-3-tier-app
I will use Python code for the AWS Lambda function. The Python code has 1 GET and 1 POST request. The GET request will retrieve a random color from DynamoDB. The POST request will add a new color to the DynamoDB table, but before it’s added, the POST request will check to see if the color already exists. If the color exists, the Lambda function will return a 403 code saying the color already exists. The Lambda function will add the color will if the color does not exist in the DynamDB table.
Step 1. Set variables in variables.tf file
Step 2. Run Terraform
terraform init terraform validate terraform plan -out=plan.out terraform apply plan.out
Step 3. Retrieve API Gateway API Key to access the API Gateway Endpoint.
API_KEY=$(terraform output -raw api_gateway_api_key) API_ENDPOINT=$(terraform output -raw url_get-shirt-color)
Step 3. Test API Gateway connectivity to AWS Lamdba and Amazon DynamoDB by running a GET request.
curl -sS -H "x-api-key: $API_KEY" "$API_ENDPOINT"